S-des Key Generation Code In C 2b 2b 4
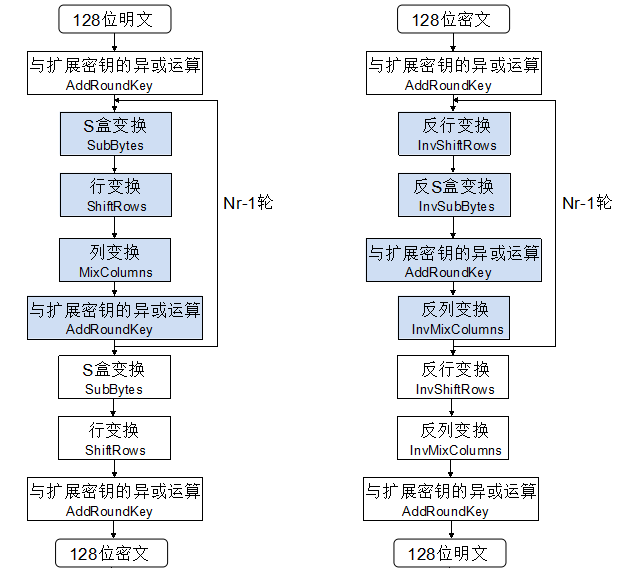
We rearrange 32bit text by following the order of that matrix. See the matrix in below code After expansion permutation we have to XOR the output 48bit with a 48bit sub key. Let see how that 48bit sub key generating from 64bit original key. Permutated Choice 1: Initially we take a 64 bit key and then apply to permutated choice 1. As we know S-DES has two round and for that we also need two keys, one key we generate in the above steps (step 1 to step 5). Now we need to generate a second bit and after that we will move to encrypt the plain text or message. It is simple to generate the second key. Simply, go in step 4 copy both halves, each one consists of 5 bits.
S-des Key Generation Code In C 2b 2b 1
|